If you want to code your website yourself and add a little fiesta, good cheer and celebration, I have the library for you.
It’s called Canvas Confetti and it allows you to add animated confetti, emojis or snow to your web pages.
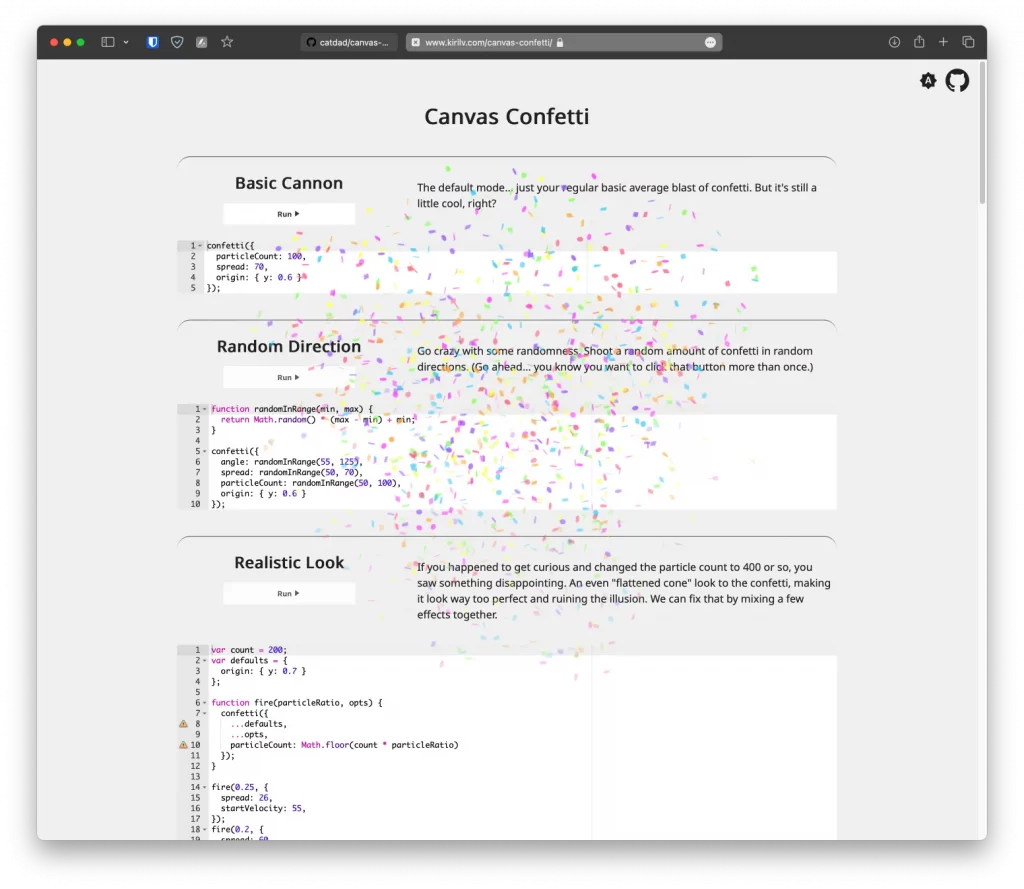
Installation couldn’t be easier:
npm install canvas-confetti
Then import the Canvas Confetti library into your JavaScript file where you want to use the confetti animation like this:
import confetti from 'canvas-confetti';
To start the confetti animation, call the function confetti()
without settings or with custom options. Here is an example of starting the default animation:
confetti();
And here is an example of starting the animation with custom options:
confetti({
particleCount: 150, // Nombre de confetti à lancer
spread: 180, // Angle maximal de dispersion des confettis
startVelocity: 30, // Vitesse initiale des confettis
origin: {
x: Math.random(), // Position initiale aléatoire des confettis sur l'axe horizontal
y: Math.random() - 0.2 // Position initiale légèrement plus élevée sur l'axe vertical
},
colors: ['#ff0000', '#00ff00', '#0000ff'], // Couleurs des confettis
shapes: ['square', 'circle', 'star'], // Formes des confettis
scalar: 2 // Taille des confettis
});
To reset the confetti animation and delete all currently animated confetti, use the method confetti.reset()
:
confetti.reset();
If you want to limit the space on your page where the confetti appears, you can use a custom canvas. To do this, create an element <canvas>
in your HTML and call the function confetti.create()
by handing him the element <canvas>
and optional global options:
const canvas = document.createElement('canvas');
document.body.appendChild(canvas);
const myConfetti = confetti.create(canvas, { // Options globales facultatives
resize: true, // Permet de redimensionner le canvas en fonction de la fenêtre
useWorker: true // Utilise un web worker pour le rendu du confetti
});
myConfetti({ // Options personnalisées
particleCount: 100,
spread: 160
// Autres options personnalisées
});
Finally, if you want to create custom confetti from an SVG shape or text, use the methods confetti.shapeFromPath
And confetti.shapeFromText
:
const pathShape = confetti.shapeFromPath({ path: 'M0 10 L5 0 L10 10z' }); // Forme SVG
const textShape = confetti.shapeFromText({ text: '🐈', scalar: 2 }); // Texte personnalisé
confetti({
shapes: [pathShape, textShape],
scalar: 2
});
It’s best to take a look the demo page, which also contains integration examples.
]